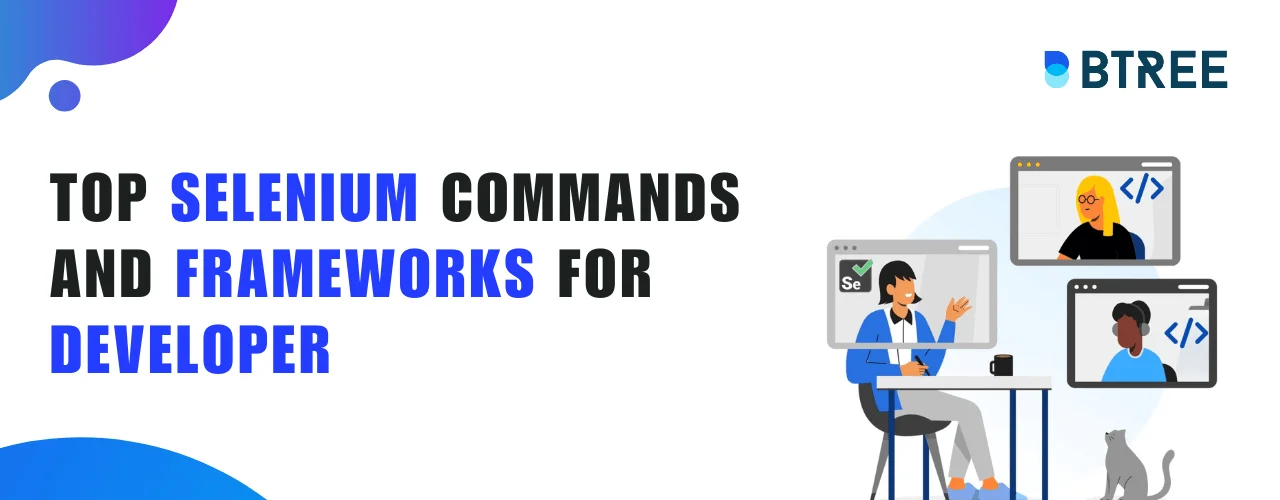
What is Selenium?
Selenium has become the most powerful open-source automation software testing tool that helps developers to test web applications across a variety of browsers and platforms. With its extensive capabilities, it empowers developers to automate browser actions and performs various functional tests without any effort. It supports many programming languages like Java, Python, and C#.
Cross-browsing is made simple by its compatibility with a variety of browsers, like Chrome, Firefox, and Safari. Additionally, Selenium’s integration with well-known testing frameworks including TestNG and JUnit enables effective test monitoring and reporting.
Whether you are an experienced developer or just beginning your path into software and browser testing, Selenium delivers an appropriate set of tools to enhance the quality of your online apps while saving valuable time and effort.
Check out our course Selenium With Python Course if you’re aiming to become one.
Throughout this article, we will examine selenium commands and frameworks that each developer has to be familiar with in 2023.
What are Selenium Commands?
Selenium commands are the actions that we perform on a web browser using Selenium. It has a wide variety of commands through which we can perform various types of actions on a web browser.
These are mainly used for interacting with a web browser and automating different tasks. They can be used for performing regression testing, exploring and testing web applications.
Now, let's discuss the types of selenium commands with suitable code and output.
The Selenium commands are mainly classified into 5 different types:
1. Navigational Selenium Commands:
These commands allow you to visit web pages while performing automated testing. Navigational commands replicate user actions such as clicking links, going to other URLs, refreshing the pages, and moving forward or backward in the browser’s history.
Below are some common navigational commands:
1. back():
This command is used to open the previous web page of the current page on a browser.
Example Code Using cricbuzz:
from selenium import webdriver web_driver = webdriver.Chrome(chrome_options=options, executable_path=, 'F:/VS Code/chromedriver.exe' ) page_url = 'https://www.cricbuzz.com/' # URL of the webpage web_driver.get(page_url) web_driver.back()
Output:
Screen 1:

Screen 2:
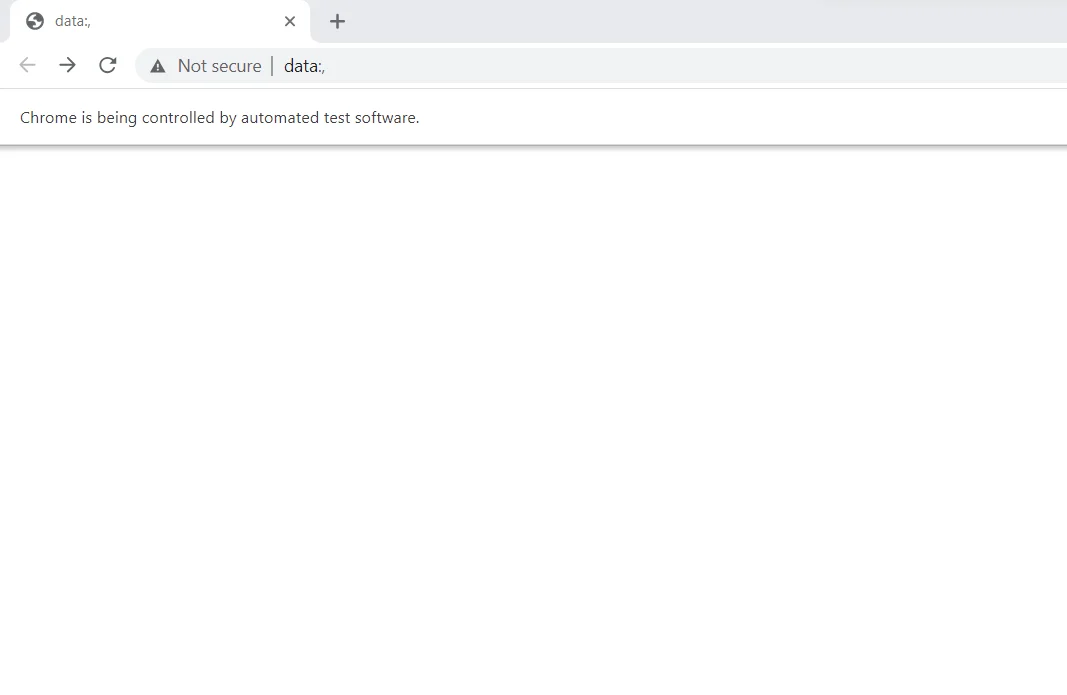
Now let's discuss the forward command which is different from the back() command.
2. forward():
This command is used to open the next web page of the current page on a browser.
Example Code Using cricbuzz Domain:
from selenium import webdriver web_driver = webdriver.Chrome(chrome_options=options, executable_path=, 'F:/VS Code/chromedriver.exe' ) # URL of the webpage page_url = 'https://www.cricbuzz.com/' web_driver.get(page_url) web_driver.back() web_driver.forward()
Screen 1:

Screen 2:
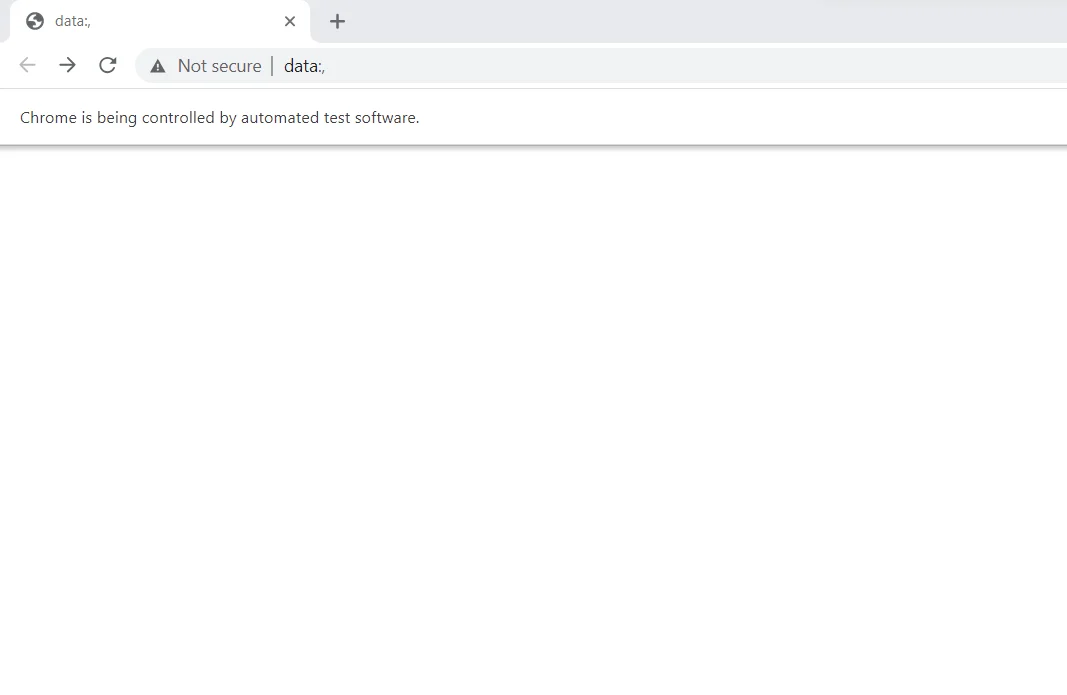
Screen 3:

So, forward() is used to move the browser forward in history. This command thus is used to get the source code of the page.
3. get_page_source():
This command is used to get the HTML source code of the page.
Example Code:
from selenium import webdriver web_driver = webdriver.Chrome(chrome_options=options, executable_path=, 'F:/VS Code/chromedriver.exe' ) # URL of the webpage page_url = 'https://www.cricbuzz.com/' web_driver.get(page_url) html_page_source = web_driver.page_source print( "Page Source:" , html_page_source)
Output:

Now we will know about some selenium commands and how they are used for web elements.
Selenium commands to search for WebElements:
These commands help you to find and interact with particular elements on a web page. These web elements are found on web pages, including buttons, links, text fields, checkboxes, etc.
Here are some common methods to search for web elements.
1. findElement():
This command is useful for searching a single web element on the page.
Example Code:
from selenium import webdriver from selenium.webdriver.common.by import By from selenium.common.exceptions import NoSuchElementException # Path of the web browser driver is saved web_driver = webdriver.Chrome(chrome_options=options, executable_path=, 'F:/VS Code/chromedriver.exe' ) page_url = 'https://www.cricbuzz.com/' try: web_driver.get(page_url) # Finding an element of web page using its ID web_driver.quit() req_element = web_driver.find_element(By.ID ,( "ad-skin" ) print( "Element found by ID:" , req_element) except NoSuchElementException: print( "No Element found." ) web_driver.quit()
Output:

Another find command is also used to find elements but multiple elements on a page, let's make a discussion on it.
findElements():
This command is used to find multiple web elements or a list of elements on the page.
Example Code:
from selenium import webdriver from selenium.webdriver.common.by import By from selenium.common.exceptions import NoSuchElementException #path of the web browser driver is saved web_driver = webdriver.Chrome(chrome_options=options, executable_path= 'F:/VS Code/chromedriver.exe' ) #path of the web browser driver is saved page_url = 'https://www.cricbuzz.com/' #URL of the webpage try: web_driver.get(page_url) # Finding an elements of web page using its name print( "Elements found by Name:" , req_element) except NoSuchElementException: print( "No Element found." ) web_driver.quit()
Output:

Now we will study how commands handle frames in Selenium web drivers.
3. Commands to handle Frames in Selenium WebDriver
In the Selenium web driver, frames are used to separate elements of a web page into multiple independent sections, Each of which has its own HTML document. These frames can be utilized to highlight various pieces of content on a single web page. During automating tests using Selenium web drivers, you might come across a situation where you need to connect/interact with elements inside frames.
For handling these frames in Selenium, you can use the below commands:
switchTo().frame():
We use this command to switch the focus of Selenium to a specific frame of the page.
from selenium import webdriver web_driver = webdriver.Chrome(chrome_options=options, executable_path= 'F:/VS Code/chromedriver.exe' ) page_url = 'https://www.google.com//' web_driver.get(page_url) # Switch to a frame by its tag name frame = web_driver.find_element(By.TAG_NAME, 'iframe' web_driver.switch_to.frame(frame) # Getting html source of the selected frame only html_page_source = web_driver.page_source print( "Page Source:" , html_page_source) web_driver.quit()
Output:
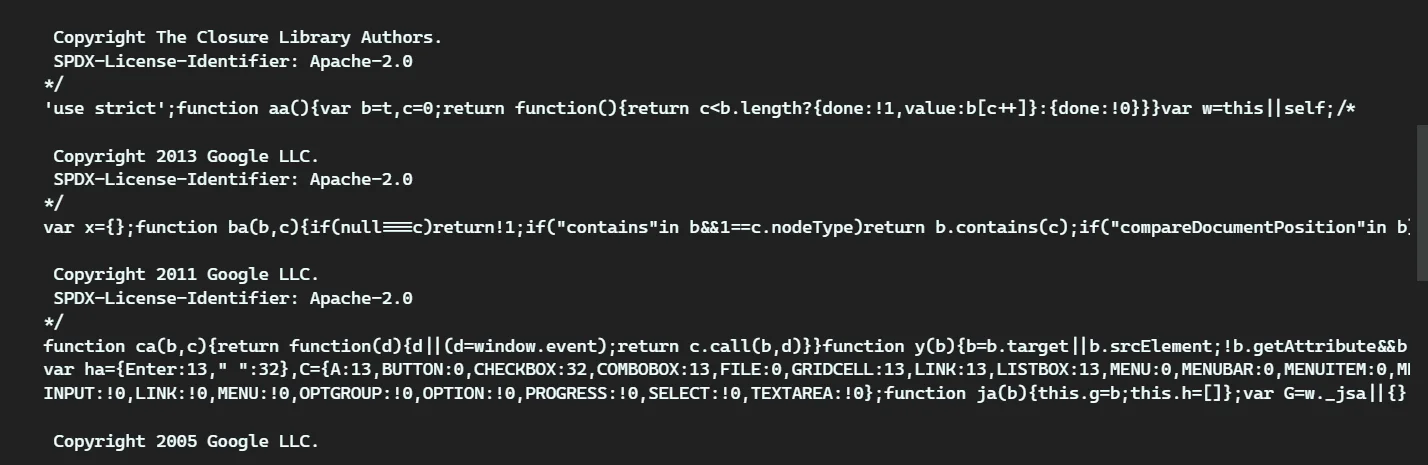
switchTo().defaultContent():
We use this command to switch back the focus of Selenium to a default frame of the page.
Example Code:
from selenium import webdriver web_driver = webdriver.Chrome(chrome_options=options, executable_path= 'F:/VS Code/chromedriver.exe' ) page_url = 'https://www.google.com//' web_driver.get(page_url) # Switch to a frame by its tag name frame = web_driver.find_element(By.TAG_NAME, 'iframe' web_driver.switch_to.frame(frame) # Switching back to default content web_driver.switch_to.default_content() # Getting html source of the selected frame only html_page_source = web_driver.page_source print "Page Source:" , html_page_source) web_driver.quit()
Output:
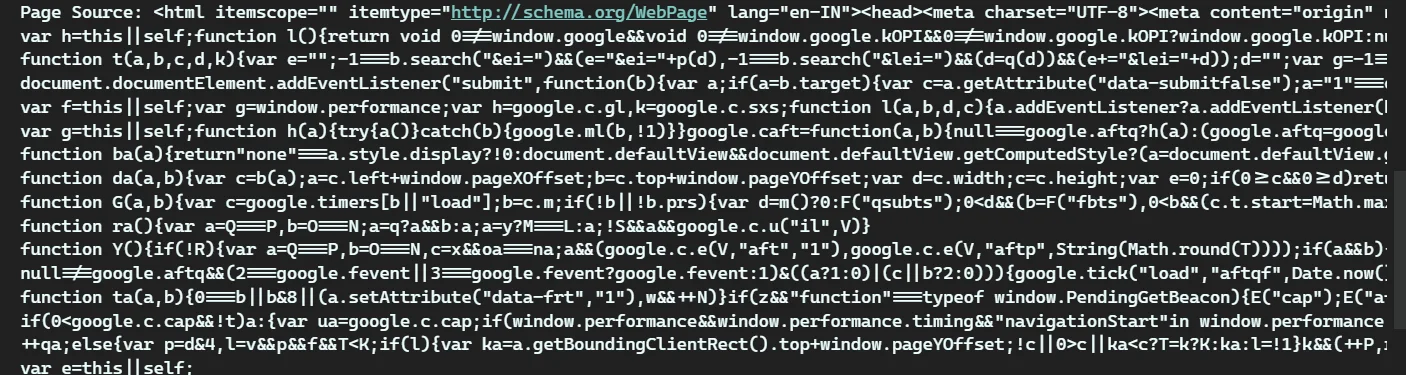
Now we will discuss a very basic and useful command which is used to get the URL of a page.
4. Basic Get() Commands:
In the Selenium web driver, the get command is used to access /open a certain URL in the browser window. It is the most basic and important command that you will use this command frequently while building automation scripts. The get method loads a tab of a website and waits for the page until fully loaded before processing the next command
get(page_url):
It opens a specified URL in the web browser.
Example Code:
# Code to use the get() function of Selenium to get the text of a web element and store it in a variable. from selenium import webdriver # URL of the webpage page_url = 'https://www.linkedin.com/company/articulate-content/' # Path of the web browser driver is saved web_driver.get(page_url) # Switch to a frame by its tag name frame = web_driver.find_element(By.TAG_NAME, 'iframe' web_driver.switch_to.frame(frame) # Switching back to default content web_driver = webdriver.Chrome(executable_path= 'F:/VS Code/chromedriver.exe' ) try: web_driver.get(page_url) input( "Press Enter to close the browser..." ) finally: web_driver.get(page_url) input( "Press Enter to close the browser..." ) web_driver.quit()
Output:
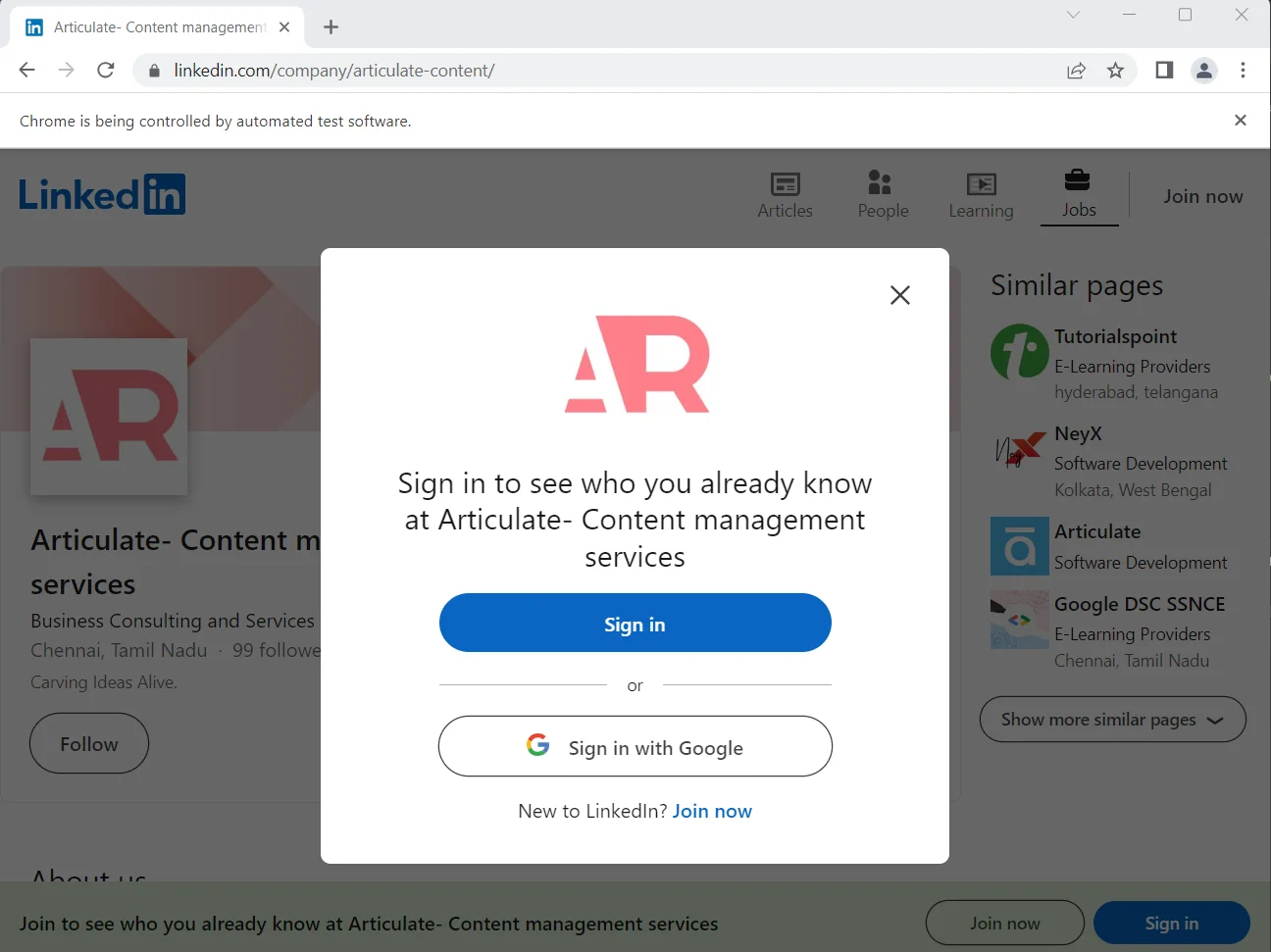
After we get there we will study about the wait command which is a command related to time.
5. Wait Commands:
In the Selenium web driver, wait commands are used to stop the execution of the script for a set amount of time until specific conditions are met. It ensures that the scripts interact with web elements only when they are ready and prevents syncing problems between the test script and the web page’s loading process.
Below mentioned are some wait commands.
1. implicitWait():
This is used to set the implicit wait time for WebDriver.
Example Code:
from selenium import webdriver from selenium.webdriver.common.by import By from selenium.common.exceptions import NoSuchElementException import time #path of the web browser driver is saved web_driver = webdriver.Chrome(chrome_options=options, executable_path= 'F:/VS Code/chromedriver.exe' ) #path of the web browser driver is saved page_url = 'https://www.cricbuzz.com/' #URL of the webpage try: # Finding an elements of web page using its name start_time = time.time() req_element = web_driver.find_elements(By.NAME, "google-signin-client_id" ) print( "Elements found by Name:" , req_element) end_time = time.time() wait_time = end_time - start_time print( "Waiting time: {:.2f} seconds" ,.format(wait_time)) except NoSuchElementException: print( "No Element found." ) web_driver.quit()
Output:

Now let's move forward with another type of wait command.
2. explicitWait():
This command waits for a specific condition to get satisfied before continuing.
Example Code:
from selenium import webdriver from selenium.webdriver.common.by import By from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC import time #path of the web browser driver is saved web_driver = webdriver.Chrome(chrome_options=options, executable_path= 'F:/VS Code/chromedriver.exe' ) page_url = 'https://www.cricbuzz.com/' #URL of the webpage try: # Wait up to 10 seconds for the element with ID 'element_id' to be clickable element = WebDriverWait(web_driver, 10).until(EC.element_to_be_clickable((By.ID, 'google-signin-client_id' ))) print( "Element found:" , element.text) try: Exception as e: print( "Not clickable within the specified time" ) web_driver.quit()
Output:

In the above portion of the article we have discussed about Selenium commands now we will move forward with the Selenium framework and their types.
Our Lovely Student feedback
What are Selenium Frameworks?
Selenium frameworks are the scripts of code that help to automate web browser testing. They provide a structure to organize our tests and they increase the code reusability and make it easier to share tests with other developers.
There are many frameworks of Selenium that are available with their own strengths and weaknesses.
Some of today’s most popular Selenium Frameworks are listed below:
1. Linear Scripting Framework
It is a fundamental test automation framework that is implemented in the form of the “Record and playback” method. It might indicate that the scripts covered inside it are run linearly. That is, they are executed sequentially in order they are generated without complicated flow control. This sort of framework is used to test apps that are of a small size.
This framework is useful in testing small-sized applications. This can be ideal for uncomplicated, step-by-step execution tasks or small automation work. In this testing technique, each test case needs its own sets of prerequisites and script executions. All the test phases in this testing process including browsing, navigation, user inputs, and setting checking points have been accurately recorded with the help of testers. They subsequently execute their tests by playing back these scripts.
2. Modular Testing Framework
It is a software testing strategy emphasizing the organization of test cases into small, more manageable, independent components. Each module focuses on testing specific components or features of the software program. Combining these separate test scripts for the creation of a bigger script by using a master script to conduct the specific situations in each case.
It helps in improving test cases' reusability, maintainability, and durability.
The many modules/components are called by this master script to guarantee end-to-end test case scenarios that are capable of being executed from beginning to end.
3. Data-Driven Framework
It is a popular testing framework that helps to separate the test script logic from the test data by accessing users/testers to run/execute the same test scripts multiple times. In this framework, test data is kept separate from the test script in external data sources including databases, XML files, Excel files, and CSV files. The test scripts use the test data which is obtained from these outside sources for carrying out test actions and approvals. Testers can simply modify this data using this method without bothering to change the test script itself.
4. Keyword-Driven Framework
It is also called a table-driven testing framework. It is a type of framework that uses keywords to drive the testing process. It focuses on separating the logic of the test script logic from the test data and test procedures. With the help of a set of keywords or action words, testers can design and execute test cases that represent particular test steps and operations.
The execution flow in this framework follows these steps:
- Firstly, the test script reads the test cases along with related test data and keywords from the test case repository.
- Then, each keyword is decoded by test script and then calls the corresponding keyword function from the library.
- The keyword function executes the assigned function on the provided data set.
- Once all the keywords are executed, the test script records the test output, whether pass or fail, and then moves on to the next keyword.
5. Page Object Model (POM):
This framework is based on the Page Object pattern. It is a powerful framework that increases the maintainability and reusability of test automation code. By organizing tests into reusable models, POM minimizes duplication and improves maintenance tasks in automated testing projects by collecting tests into reusable units. This pattern creates a separate class for each page in the application. This makes the test scripts more modular and easier to maintain.
By implementing POM, Scalability is guaranteed to increase due to the addition of new features. Overall, accepting the page Object Model allows teachers to build strong automation scripts that are more reliable across platforms and browsers, and easier to maintain over time.
6. JUnit
Junit testing framework is known for its simplicity and efficiency. It is a framework for Java applications. Junit facilitates the process of creating and running tests simpler with its easy syntax and vast functionality. By providing a structured framework to define test cases, JUnit makes sure that each section of the code is examined carefully for bugs and errors. Moreover, By providing automated testing during the build process, Junit’s seamless interface with popular development environments including Eclipse or IntelliJ IDEA helps to improve performance. It empowers programmers/testers to build reliable and trustworthy code.
7. TestNG
The full form of TestNG is Test Next Generation. It is a highly utilized framework that provides strong capabilities and functionality for automated testing. It is inspired by JUnit, which is another popular testing framework for Java.
TestNG is created to overcome some limitations Of JUnit and to provide some extra features to support more advanced testing. TestNG has grown to be a preferred alternative for test automation in the Java ecosystem because of its numerous advantages and features.
8. Cucumber
Cucumber is a BDD (Behavior-Driven Development) framework that can be used with Selenium. It is a good choice for projects that use BDD.BDD is an Agile Methodology that fosters teamwork between programmers, testers, and business stakeholders to convey a system’s performance through user stories or scenarios that are expressed in a language that is meaningful to humans.
9. Hybrid Framework
It is a combination of many different automation frameworks, including data-driven and keyword-driven frameworks, to provide adaptability. It offers flexibility by utilizing modularization, test data separation, and keyword-driven approaches to create robust and maintainable automation scripts.
10. Appium
It can be used with Selenium to automate web-based mobile applications. Making reliable test scripts is made by Appium’s user-friendly interface and broad support for numerous programming including Java, Python, and Ruby.
This was just a short introduction to the Appium, if you want to know more about it you can refer to the Official Documentation.
Conclusion
In conclusion, for modem software professionals learning Selenium is a must for web automation. It helps the testers and developers to easily automate repetitive tasks, ensure application quality and improve overall productivity. Properties of Selenium like versatility, community support, and compatibility with various languages make it a valuable skill for being competitive in the tech industry. Learning Selenium is a smart investment for software reliability enhancement and streamlining web testing.
Selenium Course Schedule
Name | Date | Details |
---|---|---|
Selenium Training |
19 Aug 2023
(Sat-Sun) Weekend Batch |
View Details |
Selenium with Python Training |
26 Aug 2023
(Sat-Sun) Weekend Batch |
View Details |
Python Training |
02 Sept 2023
(Sat-Sun) Weekend Batch |
View Details |
Looking For 100% Salary Hike?
Speak to our course Advisor Now !